《生死僵局》(Deadlock)偏移量獲取教學
☑️ 生死僵局偏移量獲取指南
本教學將介紹如何獲取生死僵局(Deadlock)遊戲中的重要偏移量,包括:
- dwEntityList
- ViewMatrix
- LocalPlayerController (LocalPlayer)
- CCameraManager
- SchemaSystemInterface
請注意,由於遊戲經常更新,這些偏移量可能會在幾小時後失效。以下提供C#和C++兩種程式碼,可以運行其中之一來獲取最新偏移量。
☑️ C#程式碼- using System;
- using System.Collections.Generic;
- using System.Diagnostics;
- using System.Linq;
- using System.Runtime.InteropServices;
- using System.Text;
-
- class Program
- {
- [StructLayout(LayoutKind.Sequential)]
- public struct MODULEINFO
- {
- public IntPtr lpBaseOfDll;
- public uint SizeOfImage;
- public IntPtr EntryPoint;
- }
-
- class Signature
- {
- public string Pattern { get; }
- public uint Offset { get; }
- public uint Extra { get; }
-
- public Signature(string pattern, uint offset, uint extra)
- {
- Pattern = pattern;
- Offset = offset;
- Extra = extra;
- }
-
- public List<byte> ParsePattern()
- {
- var bytes = new List<byte>();
- var patternParts = Pattern.Split(' ');
-
- foreach (var byteStr in patternParts)
- {
- if (byteStr == "?" || byteStr == "??")
- {
- bytes.Add(0);
- }
- else
- {
- bytes.Add(Convert.ToByte(byteStr, 16));
- }
- }
-
- return bytes;
- }
-
- static T ReadMemory<T>(IntPtr processHandle, IntPtr address) where T : struct
- {
- var size = Marshal.SizeOf(typeof(T));
- var buffer = new byte[size];
- int bytesRead;
-
- if (ReadProcessMemory(processHandle, address, buffer, size, out bytesRead) && bytesRead == size)
- {
- GCHandle handle = GCHandle.Alloc(buffer, GCHandleType.Pinned);
- T result = (T)Marshal.PtrToStructure(handle.AddrOfPinnedObject(), typeof(T));
- handle.Free();
- return result;
- }
- throw new InvalidOperationException("Failed to read memory.");
- }
-
-
- public void Find(IEnumerable<byte> memory, IntPtr processHandle, IntPtr moduleBase)
- {
- var pattern = ParsePattern();
- var printedAddresses = new HashSet<UIntPtr>();
-
- for (int i = 0; i < memory.Count() - pattern.Count(); i++)
- {
- bool patternMatch = true;
- for (int j = 0; j < pattern.Count(); j++)
- {
- if (pattern[j] != 0 && memory.ElementAt(i + j) != pattern[j])
- {
- patternMatch = false;
- break;
- }
- }
-
- if (patternMatch)
- {
- var patternAddress = (UIntPtr)(moduleBase.ToInt64() + i);
- int of = ReadMemory<int>(processHandle, (IntPtr)(patternAddress.ToUInt64() + Offset));
- var result = patternAddress.ToUInt64() + (UInt64)of + Extra;
-
- var resultPtr = new UIntPtr((UInt64)result - (UInt64)moduleBase.ToInt64());
-
- // Print the address only if it's not already printed
- if (printedAddresses.Add(resultPtr))
- {
- Console.WriteLine($"> 0x{resultPtr.ToUInt64():X}");
- }
- }
- }
- }
- }
-
- static void Main()
- {
- Console.WriteLine("By 5komar (Catrine)\n");
- Console.WriteLine("My Discord Username > catrine\n");
-
- var localPlayerSig = new Signature("48 8B 0D ? ? ? ? 48 85 C9 74 65 83 FF FF", 3, 7);
- var viewMatrixSig = new Signature("48 8D ? ? ? ? ? 48 C1 E0 06 48 03 C1 C3", 3, 7);
- var entityListSig = new Signature("48 8B 0D ? ? ? ? 8B C5 48 C1 E8", 3, 7);
- var CCameraManagerSig = new Signature("48 8D 3D ? ? ? ? 8B D9", 3, 7);
- var chemas_sig = new Signature("48 89 05 ? ? ? ? 4C 8D 0D ? ? ? ? 0F B6 45 E8 4C 8D 45 E0 33 F6", 3, 7);
-
-
- string processName = "project8.exe";
- var processHandle = GetProcessHandle(processName);
-
- if (processHandle == IntPtr.Zero)
- {
- Console.WriteLine("Game process not found!");
- return;
- }
-
- var moduleInfo = GetModuleInfo(processHandle, "client.dll");
- var moduleInfo2 = GetModuleInfo(processHandle, "schemasystem.dll");
- if (moduleInfo.lpBaseOfDll == IntPtr.Zero)
- {
- Console.WriteLine("client.dll not found!");
- return;
- }
- if (moduleInfo2.lpBaseOfDll == IntPtr.Zero)
- {
- Console.WriteLine("schemasystem.dll not found!");
- return;
- }
-
- var memory = ReadMemoryBytes(processHandle, moduleInfo.lpBaseOfDll, (int)moduleInfo.SizeOfImage);
- var memory2 = ReadMemoryBytes(processHandle, moduleInfo2.lpBaseOfDll, (int)moduleInfo.SizeOfImage);
-
- Console.WriteLine("LocalPlayerController:");
- localPlayerSig.Find(memory, processHandle, moduleInfo.lpBaseOfDll);
- Console.WriteLine("ViewMatrix:");
- viewMatrixSig.Find(memory, processHandle, moduleInfo.lpBaseOfDll);
- Console.WriteLine("EntityList:");
- entityListSig.Find(memory, processHandle, moduleInfo.lpBaseOfDll);
- Console.WriteLine("CCameraManager:");
- CCameraManagerSig.Find(memory, processHandle, moduleInfo.lpBaseOfDll);
- Console.WriteLine("SchemaSystemInterface:");
- chemas_sig.Find(memory2, processHandle, moduleInfo2.lpBaseOfDll);
-
- CloseHandle(processHandle);
- }
-
- static List<byte> ReadMemoryBytes(IntPtr processHandle, IntPtr address, int size)
- {
- var buffer = new byte[size];
- ReadProcessMemory(processHandle, address, buffer, size, out _);
- return buffer.ToList();
- }
-
- static IntPtr GetProcessHandle(string processName)
- {
- processName = processName.EndsWith(".exe") ? processName.Substring(0, processName.Length - 4) : processName;
-
- var processes = Process.GetProcessesByName(processName);
- if (processes.Length > 0)
- {
- IntPtr processHandle = OpenProcess(0x0010 | 0x0400, false, processes[0].Id);
-
- if (processHandle != IntPtr.Zero)
- {
- return processHandle;
- }
- else
- {
- Console.WriteLine($"Failed to open process handle. Error code: {Marshal.GetLastWin32Error()}");
- }
- }
- else
- {
- Console.WriteLine($"No process found with name '{processName}'");
- }
-
- return IntPtr.Zero;
- }
-
- static MODULEINFO GetModuleInfo(IntPtr processHandle, string moduleName)
- {
- MODULEINFO modInfo = new MODULEINFO();
- IntPtr[] hMods = new IntPtr[1024];
- GCHandle gch = GCHandle.Alloc(hMods, GCHandleType.Pinned);
- if (EnumProcessModules(processHandle, gch.AddrOfPinnedObject(), (uint)(hMods.Length * IntPtr.Size), out uint cbNeeded))
- {
- for (int i = 0; i < (cbNeeded / IntPtr.Size); i++)
- {
- StringBuilder szModName = new StringBuilder(1024);
- if (GetModuleBaseName(processHandle, hMods[i], szModName, szModName.Capacity) > 0)
- {
- if (moduleName == szModName.ToString())
- {
- GetModuleInformation(processHandle, hMods[i], out modInfo, (uint)Marshal.SizeOf(typeof(MODULEINFO)));
- break;
- }
- }
- }
- }
- gch.Free();
- return modInfo;
- }
-
- [DllImport("psapi.dll", SetLastError = true)]
- static extern bool EnumProcessModules(IntPtr hProcess, IntPtr lphModule, uint cb, out uint lpcbNeeded);
-
- [DllImport("psapi.dll", SetLastError = true)]
- static extern uint GetModuleBaseName(IntPtr hProcess, IntPtr hModule, StringBuilder lpBaseName, int nSize);
-
- [DllImport("psapi.dll", SetLastError = true)]
- static extern bool GetModuleInformation(IntPtr hProcess, IntPtr hModule, out MODULEINFO lpmodinfo, uint cb);
-
- [DllImport("kernel32.dll", SetLastError = true)]
- static extern bool ReadProcessMemory(IntPtr hProcess, IntPtr lpBaseAddress, [Out] byte[] lpBuffer, int dwSize, out int lpNumberOfBytesRead);
-
- [DllImport("kernel32.dll", SetLastError = true)]
- static extern bool ReadProcessMemory(IntPtr hProcess, IntPtr lpBaseAddress, out int lpBuffer, int dwSize, out int lpNumberOfBytesRead);
-
- [DllImport("kernel32.dll", SetLastError = true)]
- static extern IntPtr OpenProcess(int dwDesiredAccess, bool bInheritHandle, int dwProcessId);
-
- [DllImport("kernel32.dll", SetLastError = true)]
- static extern bool CloseHandle(IntPtr hObject);
- }
複製代碼 ☑️ C++程式碼- #include <Windows.h>
- #include <TlHelp32.h>
- #include <IOStream>
- #include <vector>
- #include <string>
- #include <Psapi.h>
- #include <sstream>
-
- struct Signature {
- std::string pattern;
- uint32_t offset;
- uint32_t extra;
-
- Signature(const std::string& pat, uint32_t off, uint32_t ext)
- : pattern(pat), offset(off), extra(ext) {}
-
- std::vector<uint8_t> parse_pattern() const {
- std::vector<uint8_t> bytes;
- std::istringstream patternStream(pattern);
- std::string byteStr;
-
- while (patternStream >> byteStr) {
- if (byteStr == "?" || byteStr == "??") {
- bytes.push_back(0);
- }
- else {
- bytes.push_back(static_cast<uint8_t>(strtol(byteStr.c_str(), nullptr, 16)));
- }
- }
- return bytes;
- }
-
- void find(const std::vector<uint8_t>& memory, HANDLE processHandle, uintptr_t moduleBase) const {
- std::vector<uint8_t> pattern = parse_pattern();
- for (size_t i = 1000000; i < memory.size(); ++i) {
- bool patternMatch = true;
- for (size_t j = 0; j < pattern.size(); ++j) {
- if (pattern[j] != 0 && memory[i + j] != pattern[j]) {
- patternMatch = false;
- break;
- }
- }
- if (patternMatch) {
- uintptr_t patternAddress = moduleBase + i;
- int32_t of;
- ReadProcessMemory(processHandle, reinterpret_cast<LPCVOID>(patternAddress + offset), &of, sizeof(of), nullptr);
- uintptr_t result = patternAddress + of + extra;
- std::cout << "+ 0x" << std::hex << (result - moduleBase) << std::endl;
- }
- }
- }
- };
-
- // Helper function to convert TCHAR to std::string or std::wstring
- #ifdef UNICODE
- std::wstring toString(const TCHAR* tcharArray) {
- return std::wstring(tcharArray);
- }
- #else
- std::string toString(const TCHAR* tcharArray) {
- return std::string(tcharArray);
- }
- #endif
-
- std::string wstringToString(const std::wstring& wstr) {
- std::string str(wstr.begin(), wstr.end());
- return str;
- }
-
- HANDLE getProcessHandle(const std::string& processName) {
- PROCESSENTRY32 processEntry;
- processEntry.dwSize = sizeof(PROCESSENTRY32);
- HANDLE snapshot = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
-
- if (Process32First(snapshot, &processEntry)) {
- do {
- std::string exeFileName = wstringToString(processEntry.szExeFile);
- if (processName == exeFileName) {
- CloseHandle(snapshot);
- return OpenProcess(PROCESS_VM_READ | PROCESS_QUERY_INFORMATION, FALSE, processEntry.th32ProcessID);
- }
- } while (Process32Next(snapshot, &processEntry));
- }
- CloseHandle(snapshot);
- return nullptr;
- }
-
-
- MODULEINFO getModuleInfo(HANDLE processHandle, const std::string& moduleName) {
- HMODULE hMods[1024];
- DWORD cbNeeded;
- MODULEINFO modInfo = { 0 };
-
- if (EnumProcessModules(processHandle, hMods, sizeof(hMods), &cbNeeded)) {
- for (unsigned int i = 0; i < (cbNeeded / sizeof(HMODULE)); i++) {
- char szModName[MAX_PATH];
- if (GetModuleBaseNameA(processHandle, hMods[i], szModName, sizeof(szModName) / sizeof(char))) {
- if (moduleName == szModName) {
- GetModuleInformation(processHandle, hMods[i], &modInfo, sizeof(modInfo));
- break;
- }
- }
- }
- }
- return modInfo;
- }
-
- std::vector<uint8_t> readMemoryBytes(HANDLE processHandle, uintptr_t address, size_t size) {
- std::vector<uint8_t> buffer(size);
- ReadProcessMemory(processHandle, reinterpret_cast<LPCVOID>(address), buffer.data(), size, nullptr);
- return buffer;
- }
-
- int main() {
- std::cerr << "By 5komar (Catrine)\n" << std::endl;
-
- Signature localPlayerSig("48 8B 0D ? ? ? ? 48 85 C9 74 65 83 FF FF", 3, 7);
- Signature viewMatrixSig("48 8D ? ? ? ? ? 48 C1 E0 06 48 03 C1 C3", 3, 7);
- Signature entityListSig("48 8B 0D ? ? ? ? 8B C5 48 C1 E8", 3, 7);
- Signature CCameraManagerSig("48 8D 3D ? ? ? ? 8B D9", 3, 7);
-
-
- std::string processName = "project8.exe";
- HANDLE processHandle = getProcessHandle(processName);
- if (!processHandle) {
- std::cerr << "Game process not found!" << std::endl;
- return 1;
- }
-
- MODULEINFO moduleInfo = getModuleInfo(processHandle, "client.dll");
-
- if (!moduleInfo.lpBaseOfDll) {
- std::cerr << "client.dll not found!" << std::endl;
- return 1;
- }
-
- std::vector<uint8_t> memory = readMemoryBytes(processHandle, reinterpret_cast<uintptr_t>(moduleInfo.lpBaseOfDll), moduleInfo.SizeOfImage);
-
- std::cout << "LocalPlayerController:" << std::endl;
- localPlayerSig.find(memory, processHandle, reinterpret_cast<uintptr_t>(moduleInfo.lpBaseOfDll));
- std::cout << "ViewMatrix:" << std::endl;
- viewMatrixSig.find(memory, processHandle, reinterpret_cast<uintptr_t>(moduleInfo.lpBaseOfDll));
- std::cout << "EntityList:" << std::endl;
- entityListSig.find(memory, processHandle, reinterpret_cast<uintptr_t>(moduleInfo.lpBaseOfDll));
- std::cout << "CCameraManager:" << std::endl;
- CCameraManagerSig.find(memory, processHandle, reinterpret_cast<uintptr_t>(moduleInfo.lpBaseOfDll));
-
- CloseHandle(processHandle);
- return 0;
- }
複製代碼 ☑️ 使用說明
1. 選擇C#或C++程式碼,複製到您的開發環境中。
2. 確保您已安裝必要的開發工具和相關函式庫。
3. 編譯並運行程式。
4. 程式會自動搜索生死僵局遊戲進程並獲取所需的偏移量。
5. 獲取到的偏移量將顯示在控制台輸出中。
以下廣告滑動後還有帖子內容
☑️ 常見問題
Q1: 為什麼我無法獲取偏移量?
A1: 請確保遊戲正在運行,且您擁有足夠的權限訪問遊戲進程。
Q2: 獲取到的偏移量多久更新一次?
A2: 建議每次遊戲更新後重新運行程式獲取最新偏移量。
Q3: 使用這些偏移量是否安全?
A3: 使用任何非官方工具都有風險,請謹慎使用並自行承擔後果。
👉 GM後台版 遊戲 推薦 ⬇️⬇️⬇️ 快速玩各種二次元動漫手遊app
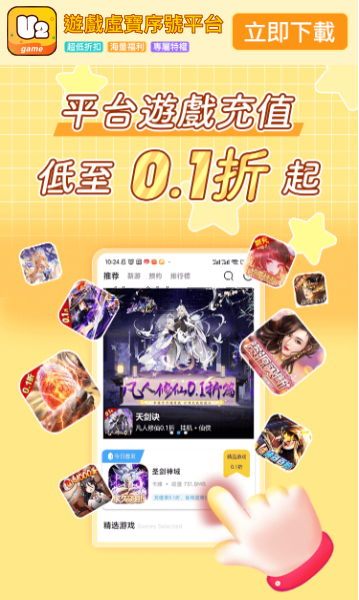
|